5 min to read
Difference Between is and ==
Understand when to use is and == in Python
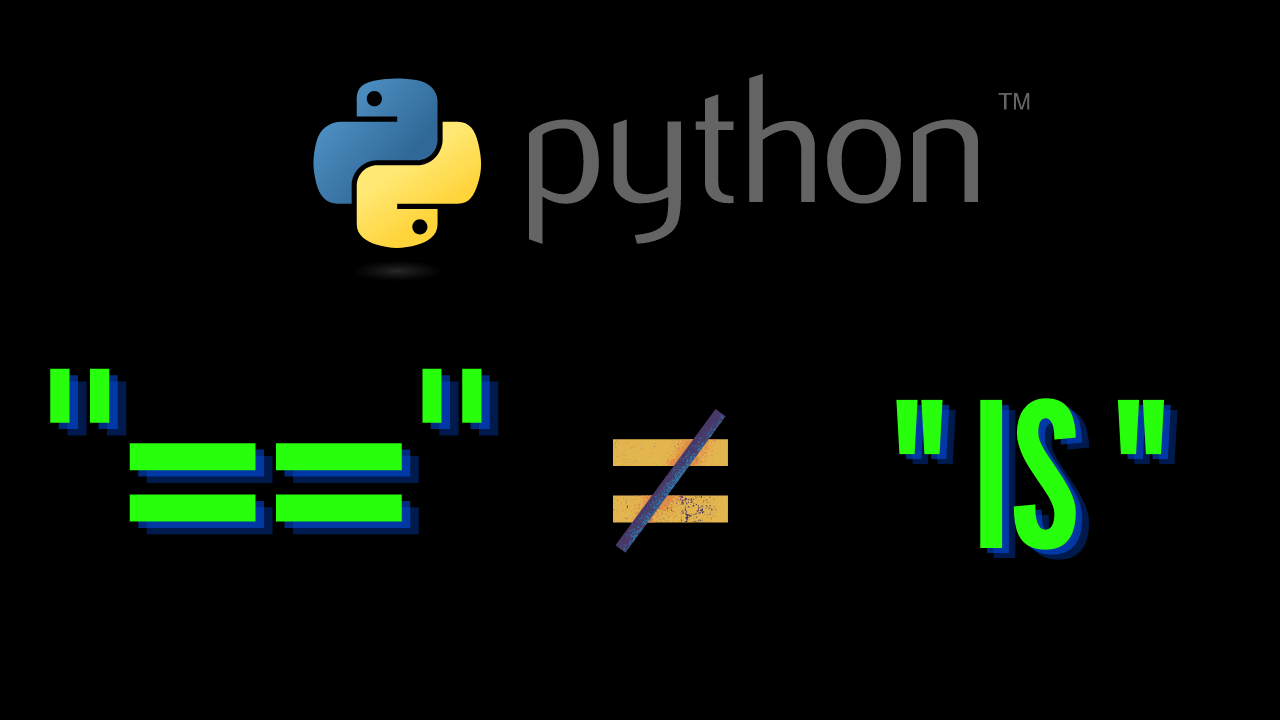
Difference Between is and ==
This is a simple tutorial of when to use is
and ==
in Python
Check out this YouTube link for more details:
Definition
==
is for value equality. It’s used to know if two objects have the same value.
is
is for reference equality. It’s used to know if two references refer (or point) to the same object, i.e if they’re identical. Two objects are identical if they have the same memory address.
Simplification
==
checks if value is equal or not and is
checks if they are exact same object
Let’s understand using id()
id()
is a built-in function in Python. It accepts a single parameter and is used to return the identity of an object.
Complete Code: Github Link
Float
a = 1.0
b = 1.0
c = 1
print(id(a))
print(id(b))
print(id(c))
print("\nFor Float:")
print(a==b)
print(a is b)
print("\nFor Float and Integer:")
print(a==c)
print(a is c)
Result:
1885460600320
1885460600008
140722084511264
For Float:
True
False
For Float and Integer:
True
False
List
a = [1,2,3,4]
b = [1,2,3,4]
print(id(a))
print(id(b))
print("--------")
print(a==b)
print(a is b)
Result:
1885462242952
1885462242824
--------
True
False
For Same Object:
a = [1,2,3,4]
b = a
print(id(a))
print(id(b))
print("--------")
print(a==b)
print(a is b)
Result:
1885461966664
1885461966664
--------
True
True
b[0] = 34
print(a)
print("--------")
print(a==b)
print(a is b)
Result:
[34, 2, 3, 4]
--------
True
True
c = a[:]
print(id(a))
print(id(c))
print("--------")
print(a==c)
print(a is c)
Result:
1885461966664
1885461989512
--------
True
False
Integer
a = 10
b = 10
print(id(a))
print(id(b))
print("--------")
print(a==b)
print(a is b)
a = -1
b = -1
print(id(a))
print(id(b))
print("--------")
print(a==b)
print(a is b)
Result:
140722084511552
140722084511552
--------
True
True
140722084511200
140722084511200
--------
True
True
Now Why is that? Let’s try for bigger numbers.
a = 257
b = 257
print(id(a))
print(id(b))
print("--------")
print(a==b)
print(a is b)
a = -6
b = -6
print(id(a))
print(id(b))
print("--------")
print(a==b)
print(a is b)
Result:
1885461868176
1885461868208
--------
True
False
1885461867568
1885461867920
--------
True
False
Integer Explanation
The current implementation keeps an array of integer objects for all integers between -5
and 256
. When you create an int in that range you actually just get back a reference to the existing object.
String
a = "Subcribe @HarshMittalYoutube"
b = "Subcribe @HarshMittalYoutube"
c = a
print(id(a))
print(id(b))
print("--------")
print(a==b)
print(a is b)
print("--------")
print(a==c)
print(a is c)
Result:
1885462270320
1885462270800
--------
True
False
--------
True
True
Complete Code: Github Link
Comments