5 min to read
Git Basic Tutorial
Learn git the right way
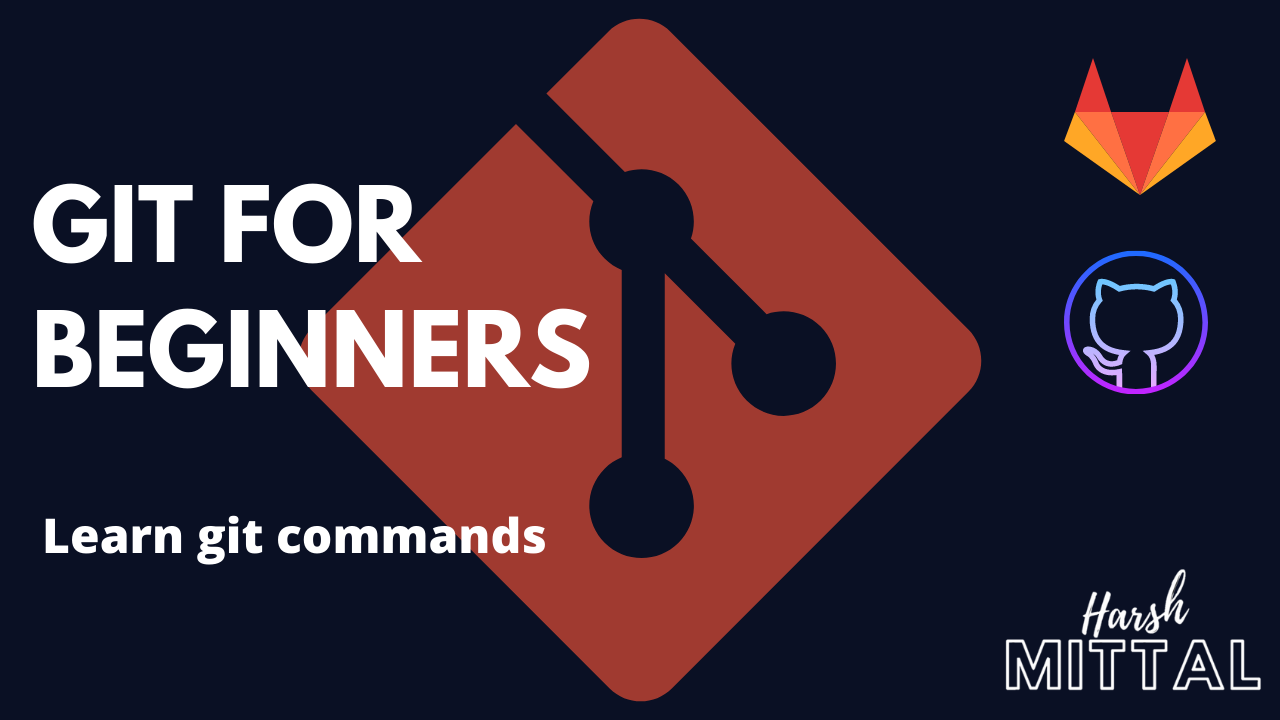
Git is software for tracking changes in any set of files, usually used for coordinating work among programmers collaboratively developing source code during software development. Its goals include speed, data integrity, and support for distributed, non-linear workflows. [1]
I have been using Git since my college projects and have helped me to organize my projects and resolve a lot of bugs. Through this blog, I will try to explain the basic concept of git that has helped me.
Contents
Git Setup
Installation
To install in Linux:
$ sudo apt install git
To install in Windows:
Download the Windows Git Intaller
To install in Mac:
Download the Mac Git Intaller
Verify Git Installation
Run:
$ git --version
Output:
git version <version_name>
Configure your Git
Before using git you need to configure it properly.
$ git config --global user.name "Harsh Mittal"
$ git config --global user.email "harshmittal2210@gmail.com"
Basic Commands
These are some of the most common git commands used.
Initialize Git
To initialize git in a folder:
git init
Add Origin
Origin is nothing but link to external git repository where your code is maintained.
Use the following command to add origin
to existing git folder:
git remote add origin <link.git>
## Example:
git remote add origin https://github.com/harshmittal2210/youtubeProjects.git
Ream more: Playing with Origins
Cloning
Cloning is nothing but downloading a copy of the project from any external git repository.
git clone https://github.com/harshmittal2210/youtubeProjects
Add Files
Add Files which will later be committed:
git add <file_name>
You can also add everything by using:
git add .
# or
git add --all
Commit changes
git commit -m "<Commit Message>"
To commit changes with description:
git commit
This will open up a text editor (customizable via git config
) asking for a commit log message, along with a list of what’s being committed:
# Please enter the commit message for your changes. Lines starting
# with '#' will be ignored, and an empty message aborts the commit.
# On branch main
# Changes to be committed:
# (use "git reset HEAD ..." to unstage)
#
#modified: Readme.md
Push Changes
Push Commits to Repository:
git push origin <branch_name>
## Example
git push origin master
New Branch
Create new branch in git
git branch dev && git checkout dev
## OR
git checkout -b dev
Change Branch
git checkout <branch_name>
## Example
git checkout dev
Playing with Origins
In Git, “origin” is a shorthand name for the remote repository that a project was originally cloned from. More precisely, it is used instead of that original repository’s URL - and thereby makes referencing much easier.
Add Multiple Origins
git remote add <origin_name> <repo_link>
## Example
git remote add origin https://github.com/harshmittal2210/youtubeProjects.git
git remote add diff_origin https://github.com/harshmittal2210/mulitCopyPaste.git
Change Existing Git origin URL
git remote set-url origin <new_link>
Git Submodules
Submodules are used when you want to use any other library within your project. It is used in such cases where you want to use both of them while keeping them separate.
Add Submodule
git submodule add <Repo_Link>
## Example
git submodule add https://github.com/harshmittal2210/mulitCopyPaste.git
Update All Submodule
This is used when you have cloned a project and you want to update all the submodules as well.
git submodule update --init --recursive
Review Pull Request
Let’s say you are working on a really big project with multiple developers. One of the developers creates a PR and you have to review it. To run the code you need to have it in your system and since it is not yet merged you cannot directly access it using a simple git pull.
The way that I prefer is using git fetch and checkout the pull request in headless mode so that unnecessary local branches are not created.
git fetch upstream pull/<ID>/head && git checkout FETCH_HEAD
Here ID is the PR ID that you can see on the pull request page.
Stash
git stash
saves all the changes you have made temporarily so that you can do some other work and come back to those changes and apply it.
$ git status
On branch main
Changes to be committed:
new file: test.py
Changes not staged for commit:
modified: Readme.md
$ git stash
Saved working directory and index state WIP on main: 7003d57 our new homepage
HEAD is now at 7003d57 our new homepage
$ git status
On branch main
nothing to commit, working tree clean
Bring back the stash:
- Apply and remove from stash
git stash pop
- Apply and keep in stash
git stash apply
Comments